Generate New Table With Foreign Key Rails
Adds a new foreign key. Fromtable is the table with the key column, totable contains the referenced primary key. The foreign key will be named after the following pattern: fkrails. You're going to have to generate your migration first to build the guardianusers table. You can read up on migrations from the rails docs and doing FK's. You will also need to associate any models that represent the two of these entities with things like hasmany or belongsto. “ It's been a long road.perhaps it is time. ” Updated December 16th, 2019. Table Relationships. Thus far in this book, all the work we've done has been with a single database table. The majority of databases you'll work with as a developer will have more than one table, and those tables will be connected together in various ways to form table relationships.
SQL FOREIGN KEY Constraint
A FOREIGN KEY is a key used to link two tables together.
A FOREIGN KEY is a field (or collection of fields) in one table that refers to the PRIMARY KEY in another table.
The table containing the foreign key is called the child table, and the table containing the candidate key is called the referenced or parent table.
Look at the following two tables:
'Persons' table:
PersonID | LastName | FirstName | Age |
---|---|---|---|
1 | Hansen | Ola | 30 |
2 | Svendson | Tove | 23 |
3 | Pettersen | Kari | 20 |
'Orders' table:
OrderID | OrderNumber | PersonID |
---|---|---|
1 | 77895 | 3 |
2 | 44678 | 3 |
3 | 22456 | 2 |
4 | 24562 | 1 |
Notice that the 'PersonID' column in the 'Orders' table points to the 'PersonID' column in the 'Persons' table.
The 'PersonID' column in the 'Persons' table is the PRIMARY KEY in the 'Persons' table.
The 'PersonID' column in the 'Orders' table is a FOREIGN KEY in the 'Orders' table.
The FOREIGN KEY constraint is used to prevent actions that would destroy links between tables.
The FOREIGN KEY constraint also prevents invalid data from being inserted into the foreign key column, because it has to be one of the values contained in the table it points to.
SQL FOREIGN KEY on CREATE TABLE
The following SQL creates a FOREIGN KEY on the 'PersonID' column when the 'Orders' table is created:
Setup ssh gitlab. I would recommend a second key, for now without passphrase: ssh-keygen -t rsa -C 'youremail@example.com' -P ' -q -f /.ssh/gitlabrsaThat will create (without any prompt) /.ssh/gitlabrsa (private key) and /.ssh/gitlabrsa.pub (public key)You need to.Navigate to the 'SSH Keys' tab in your 'Profile Settings'. Paste your key in the 'Key' section and give it a relevant 'Title'.Then add a /.ssh/config file with: Host gitlabrsaHostName gitlab.comUser gitPreferredAuthentications publickeyIdentityFile /home//.ssh/gitlabrsaFinally, you can clone any GitLab repo as your second identity with: git clone gitlabrsa:/That will be replaced automatically with git@gitlab.com:/ and will use your second key.
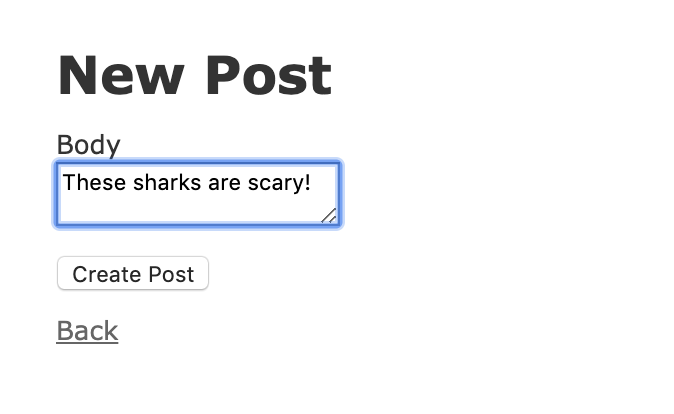
MySQL:
OrderID int NOT NULL,
OrderNumber int NOT NULL,
PersonID int,
PRIMARY KEY (OrderID),
FOREIGN KEY (PersonID) REFERENCES Persons(PersonID)
);
SQL Server / Oracle / MS Access:
OrderID int NOT NULL PRIMARY KEY,
OrderNumber int NOT NULL,
PersonID int FOREIGN KEY REFERENCES Persons(PersonID)
);
To allow naming of a FOREIGN KEY constraint, and for defining a FOREIGN KEY constraint on multiple columns, use the following SQL syntax:
MySQL / SQL Server / Oracle / MS Access:
OrderID int NOT NULL,
OrderNumber int NOT NULL,
PersonID int,
PRIMARY KEY (OrderID),
CONSTRAINT FK_PersonOrder FOREIGN KEY (PersonID)
REFERENCES Persons(PersonID)
);
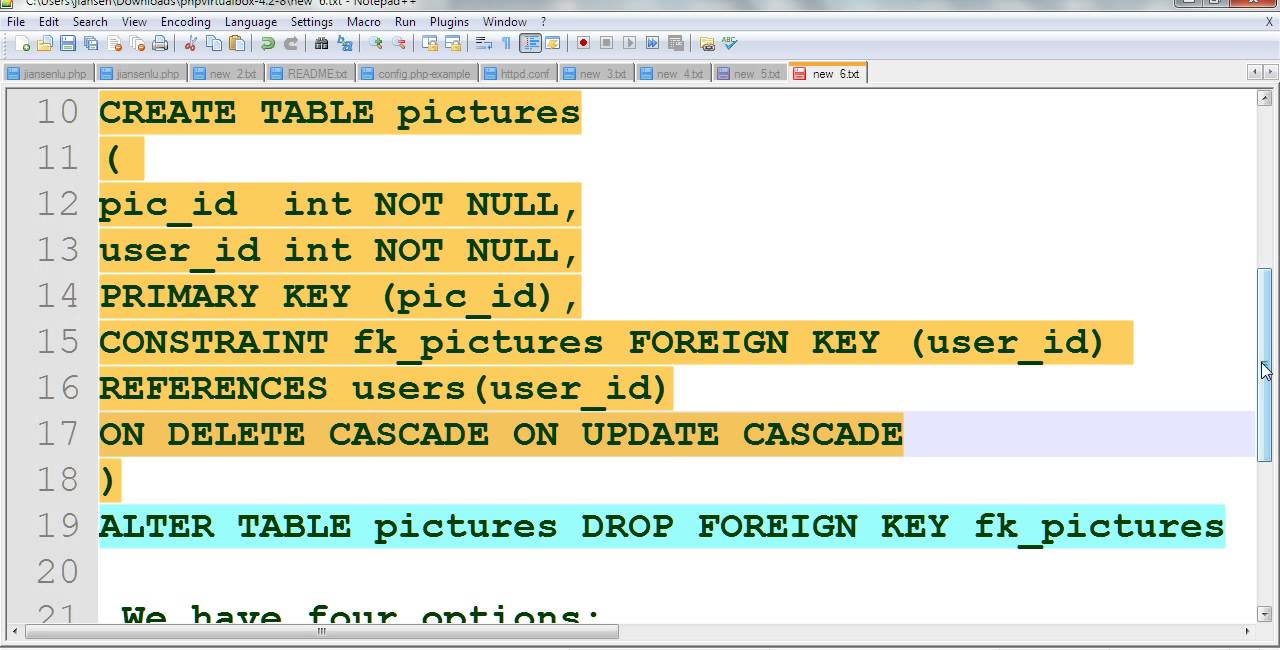
SQL FOREIGN KEY on ALTER TABLE
To create a FOREIGN KEY constraint on the 'PersonID' column when the 'Orders' table is already created, use the following SQL:
MySQL / SQL Server / Oracle / MS Access:
ADD FOREIGN KEY (PersonID) REFERENCES Persons(PersonID);
To allow naming of a FOREIGN KEY constraint, and for defining a FOREIGN KEY constraint on multiple columns, use the following SQL syntax:
MySQL / SQL Server / Oracle / MS Access:
ADD CONSTRAINT FK_PersonOrder
FOREIGN KEY (PersonID) REFERENCES Persons(PersonID);
DROP a FOREIGN KEY Constraint
To drop a FOREIGN KEY constraint, use the following SQL:
MySQL:
Rails Generate View
DROP FOREIGN KEY FK_PersonOrder;
SQL Server / Oracle / MS Access:
Rails Generate Model Foreign Key
DROP CONSTRAINT FK_PersonOrder;