Key Value Iteration In For Loop With Generator
<?php
class myIterator implements Iterator {
private $position = 0;
private $array = array(
'firstelement',
'secondelement',
'lastelement',
);
public function __construct() {
$this->position = 0;
}
public function rewind() {
var_dump(__METHOD__);
$this->position = 0;
}
public function current() {
var_dump(__METHOD__);
return $this->array[$this->position];
}
public function key() {
var_dump(__METHOD__);
return $this->position;
}
public function next() {
var_dump(__METHOD__);
++$this->position;
}
public function valid() {
var_dump(__METHOD__);
return isset($this->array[$this->position]);
}
}
$it = new myIterator;
foreach($it as $key => $value) {
var_dump($key, $value);
echo 'n';
}
?>
Java HashMap: How to get a key and value by index? HashMaps don't keep your key/value pairs in a specific order. They are ordered based on the hash that each key. Each iteration of the foreach loop calls the iterator method. When a yield return statement is reached in the iterator method, expression is returned, and the current location in code is retained. Execution is restarted from that location the next time that the iterator function is called. You can use a yield break statement to end the iteration. Nov 22, 2018 You can use an iterator to get the next value or to loop over it. Once, you loop over an iterator, there are no more stream values. Iterators use the lazy evaluation approach. Many built-in classes in Python are iterators. Microsoft key generator free download. A generator function is a function which returns an iterator. A generator expression is an expression that returns an iterator.
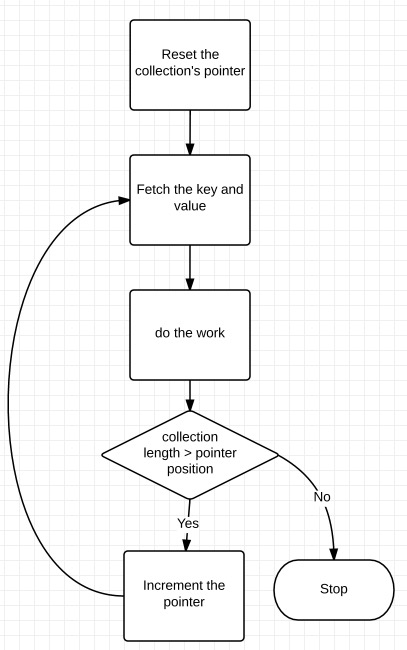
Key Value Iteration In For Loop With Generator Diagram
Add setting for generators to pass for(key = value in collection) to generator without transformation. That will allow effective target-specific implementation. Being able to generate for(key = value as foreach on PHP will make iterating over collections and map/filter operations 2-5 times faster. For Loops and Iterations A For Loop is a method of iterating through a string, list, dictionary, data frame, series, or anything else that you would like to iterate through. To iterate means to go through an item that makes up a variable.
Generate public certificate from private key. What to do now? .A lost SSH public-key or a web service generates an SSH key but does not provide the public-key part to you.